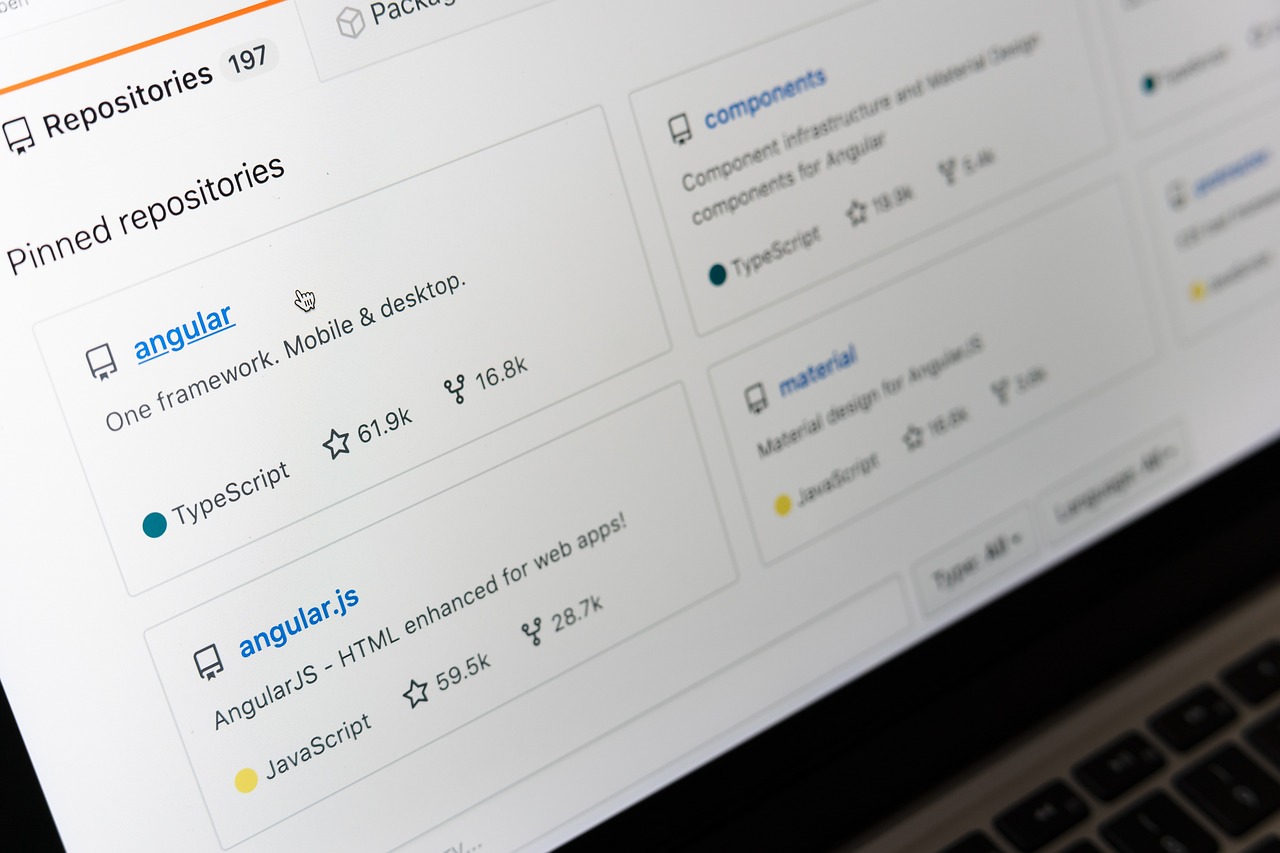
GitHub is a powerful platform for hosting and managing code, and the Octokit/rest library is a great tool for interacting with the GitHub API using Node.js. In this blog post, we will explore how to use Octokit/rest to update the content of public/private repo on GitHub. First, you will need to install the Octokit/rest library. This can be done by running the following command in your terminal:
npm install @octokit/rest
Once you have the library installed, you can start using it in your Node.js application. Here is an example of how to update a file on a GitHub repository:
import { Octokit } from '@octokit/rest';
const octokit = new Octokit({
auth: 'YOUR_GITHUB_TOKEN'
});
Generate your GitHub token from your GitHub account developer page. Follow this instruction to generate a Github access token.
const owner = 'OWNER_OF_REPOSITORY';
const repo = 'REPOSITORY_NAME';
const path = 'PATH_TO_FILE';
const message = 'Update file';
const content = new Buffer("new file content").toString('base64');
const sha = 'CURRENT_SHA_OF_FILE';
octokit.repos.updateFile({
owner,
repo,
path,
message,
content,
sha
}).then(response => {
console.log(response);
}).catch(error => {
console.log(error);
});
Explanation:
Owner: Set the repository owner of the repository.
Repo: Put the repository name properly.
Path: Set the proper path with the file name. For example, you want to update the content of the main.ts file and main.ts is available in the src folder. Then you need to set src/main.ts on the path option. If you just set main.ts, not append src/ before, then the file will not available on the root of the repository. It will create a new main.ts file on the root. So use the proper path with the file name, otherwise, it will create a new file or get an error while updating the file.
Message: It’s a commit message. So put your commit before updating.
Content: Github always saves content in base64 format (Check). When you save content through API you also need to convert your content into base64 format and then send the update request. If the new Buffer is not working then use bota to convert your data into base64. btoa(“new file content”);
Sha: If the file already exists in your GitHub repository, there will be a SHA number on this file. Whenever you update this file, You need to send the current SHA number. If you want to create a new file then you don’t need to set the SHA number because the new file doesn’t have a SHA number. But If your path already exists on the github repository then you can’t create this file. You can just update this file.
In this example, we are using the updateFile method provided by the Octokit/rest library to update a file on a GitHub repository. The method takes several parameters, including the repository owner, repository name, file path, commit message, new file content, and current SHA of the file.
Once the method is called, it will return a promise that resolves with the response from the GitHub API. In this case, the response will contain information about the updated file, including the new SHA.
It’s important to note that to update a file on GitHub, you will need to have the appropriate permissions for the repository. Additionally, you will need to provide a GitHub token for authentication.
In conclusion, updating content on GitHub using the Octokit/rest library is a simple and easy process. By following the steps outlined in this blog post, you can quickly and easily update files on a GitHub repository using Node.js.