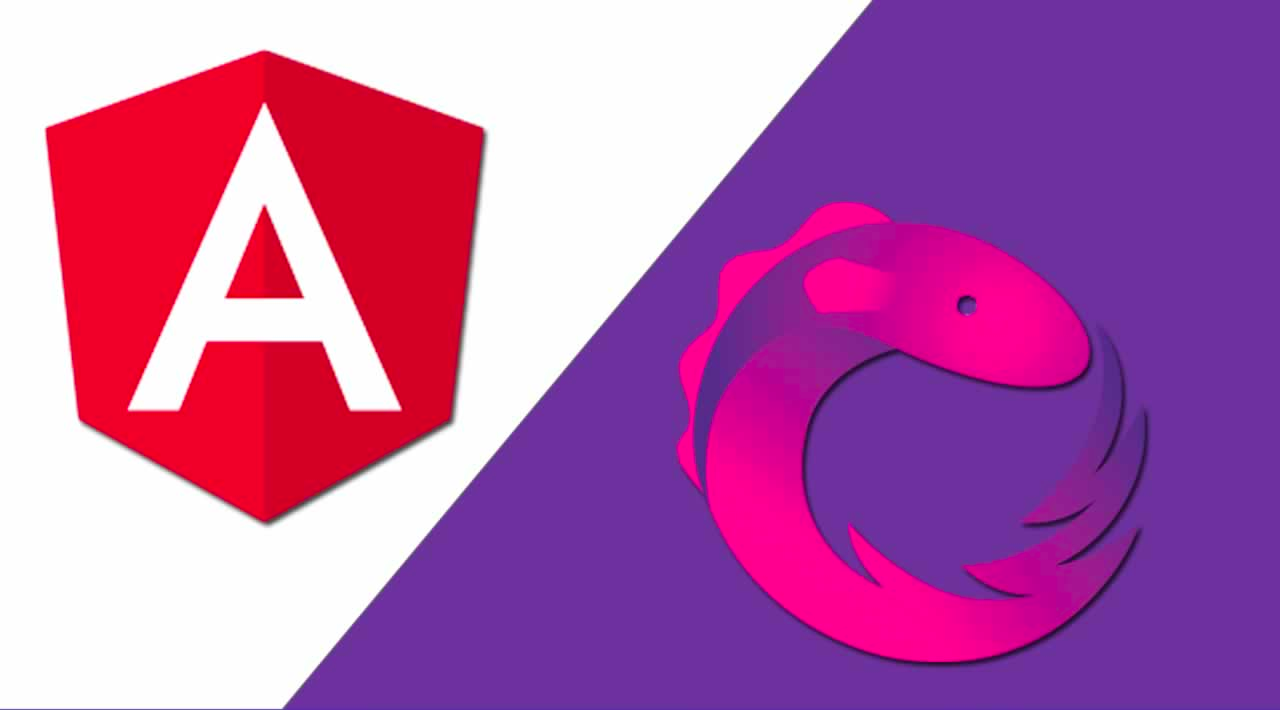
When working with Angular and RxJS, it’s important to know how to cancel previous HTTP requests. This is especially important when working with large data sets, as it can help prevent unnecessary server load and improve the overall performance of your application.
In this blog article, we’ll take a look at how to use RxJS to cancel previous HTTP requests in Angular. We’ll cover the following topics:
- Understanding the problem of multiple HTTP requests
- Using the takeUntil operator to cancel previous requests
- Creating a custom operator to cancel previous requests
Understanding the problem of multiple HTTP request
When working with Angular and RxJS, it’s common to make multiple HTTP requests to the server. However, these requests can sometimes overlap, causing unnecessary server load and slowing down the application.
For example, imagine that you have a search bar that allows users to search for products. Every time the user types in a new letter, a new HTTP request is sent to the server to retrieve the updated search results. If the user types quickly, multiple requests may be sent to the server before the previous requests have been completed.
To prevent this from happening, we need a way to cancel previous requests when a new request is made.
Using the takeUntil operator to cancel previous requests
One way to cancel previous requests is to use the takeUntil operator. The takeUntil operator takes an observable as an argument, and will unsubscribe from the source observable when the argument observable emits a value. Here’s an example of how to use the takeUntil operator to cancel previous requests in Angular:
import { takeUntil } from 'rxjs/operators';
import { Subject } from 'rxjs';
cancelSubject = new Subject();
search(term: string) {
this.cancelSubject.next(); // Cancel previous requests
this.cancelSubject.complete(); // Complete the subject
this.cancelSubject = new Subject(); // Create a new subject
// Make the HTTP request
this.http.get(`/search?term=${term}`)
.pipe(takeUntil(this.cancelSubject))
.subscribe(results => {
// Handle the results
});
}
In this example, we’re using a subject to cancel previous requests. Every time a new search is made, we call the next() method on the cancelSubject to cancel any previous requests. We then complete the subject and create a new one, so that we can cancel future requests as well.
Finally, we’re using the takeUntil operator to unsubscribe from the HTTP request when the cancelSubject emits a value.
Creating a custom operator to cancel previous requests
Another way to cancel previous requests is to create a custom operator. This can be useful if you need to cancel requests in multiple places in your application.
Here’s an example of how to create a custom operator to cancel previous requests:
import { OperatorFunction } from 'rxjs';
import { Subject } from 'rxjs';
function cancelPrevious<T>(): OperatorFunction<T, T> {
// Create a subject that will be used to cancel previous requests
const cancelSubject = new Subject();
return (source: Observable<T>) => {
cancelSubject.next(); // Cancel previous requests
cancelSubject.complete(); // Complete the subject
cancelSubject = new Subject(); // Create a new subject
return source.pipe(takeUntil(cancelSubject));
}
}