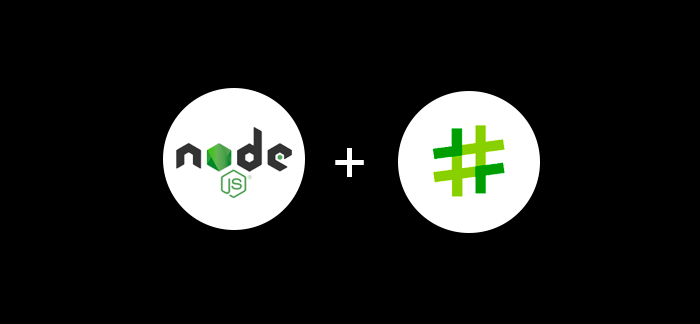
As websites and web applications continue to grow in size, it’s becoming increasingly important to optimize images for faster loading times. One way to do this is by reducing the size of the images being used. While there are a number of image optimization tools available, the Sharp npm package is a particularly useful solution for Node.js developers. In this blog post, we’ll explore how to use Sharp to reduce image size on Node.js.
Sharp is a high-performance image processing library that offers a number of features, including image resizing and compression. To use Sharp, you first need to install it in your project using npm. You can do this by running the following command in your terminal:
npm install sharp
Once you have Sharp installed, you can start using it to reduce image size. Here’s an example of how you could use Sharp to resize an image and compress it at the same time:
const sharp = require('sharp');
sharp('input.jpg')
.resize({ width: 400, height: 400, fit: 'contain' })
.toFormat('jpeg', { quality: 80 })
.toFile('output.jpg', (err, info) => {
if (err) {
console.error(err);
} else {
console.log(info);
}
});
In this example, we’re using Sharp to resize an input image to a width and height of 400 pixels, while also fitting the image inside a contain box. We then save the image as a JPEG file with a quality of 80 (which is the default quality for Sharp). The resulting image will be much smaller in size than the original, making it much faster to load for users.
Sharp also supports other image formats, including PNG, TIFF, and WebP. You can use the .toFormat() method to specify the desired format, along with any options that you want to pass along (such as quality, as in the example above).
While resizing and compressing images is a great way to optimize them for faster loading times, it’s also important to use other techniques to further optimize your images. For example, you may want to consider using lazy loading, serving images in multiple resolutions, or using next-gen image formats like WebP.
In conclusion, the Sharp npm package is a powerful tool for reducing image size on Node.js. By using Sharp to resize and compress your images, you can ensure that your web pages and applications load quickly and smoothly for your users. If you’re looking for a simple and effective way to optimize your images, give Sharp a try!